Adding Structured Data to Your Nuxt Pages
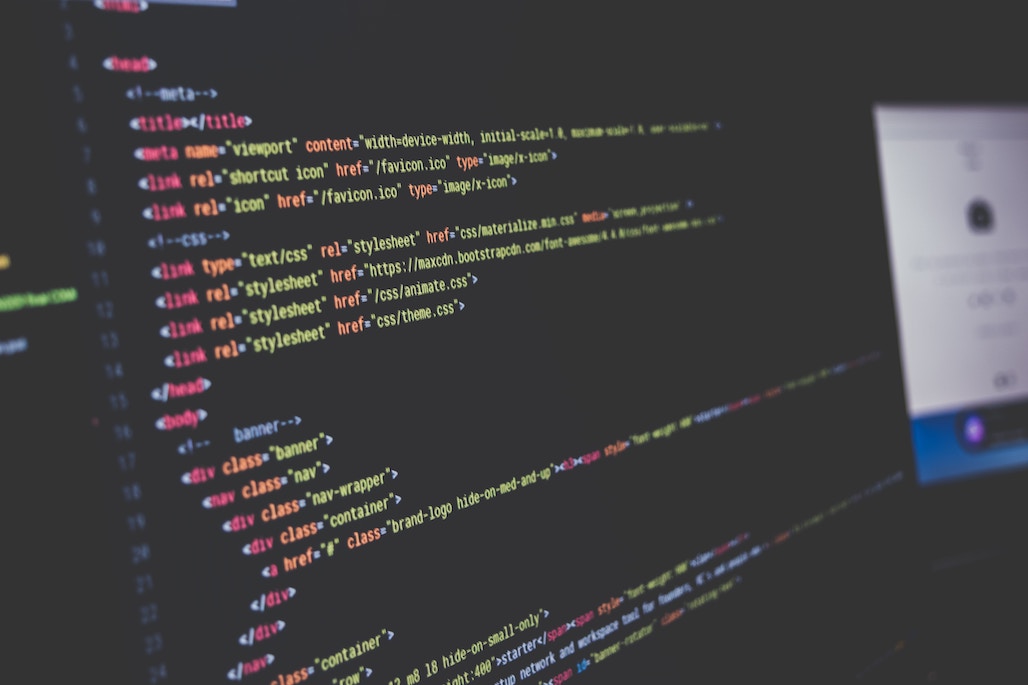
Photo by Sai Kiran Anagani on Unsplash
Structured data helps Google Search understand the content of your pages better. It's a standardised format for providing information about a page and classifying the page content.
You can add structured data by using the JSON-LD format. Below is an example of a structured data for a simple article:
<script type="application/ld+json">
{
"@type": "Article",
"headline": "Article headline",
"image": "https://example.com/photos/1x1/photo.jpg",
"datePublished": "2015-02-05T08:00:00+08:00",
"dateModified": "2015-02-05T09:20:00+08:00"
}
</script>
There are other properties that you can specify in the structured data depending on the type of content. For instance, a recipe content type could have cookTime
and recipeInstructions
.
Adding structured data via the head method
I decided to add the structure data inside the <head>
section of the page so naturally I looked into the head method, which uses vue-meta.
Since I was adding a script tag, I ended up with the following (trimmed for brevity):
<script>
export default {
head () {
const dateCreated = new Date(this.blog.created.seconds * 1000)
const dateChanged = new Date(this.blog.changed.seconds * 1000)
const structuredData = {
'@type': 'Article',
datePublished: dateCreated.toISOString(),
dateModified: dateChanged.toISOString(),
headline: this.blog.title,
image: this.blog.imageUrl
}
return {
script: [
{
type: 'application/ld+json',
json: structuredData
}
]
}
}
}
</script>
First, we construct the structured data from the blog data and add it into the script property. Luckily, Vue Meta supports adding JSON objects into script tags since version 2.1.
You can see the full source code from the repo.
Pre-Vue Meta 2.1
If for some reason you are stuck with an older version of Vue Meta, you can still inject custom code into the script tag inside the head of your page with the use of __dangerouslyDisableSanitizers
or __dangerouslyDisableSanitizersByTagID
.
Example of how the earlier code will be adjusted:
return {
__dangerouslyDisableSanitizers: ['script'],
script: [
{
type: 'application/ld+json',
innerHTML: JSON.stringify(structuredData)
}
]
}
Base from the name of these properties, it goes without saying that their use is highly discouraged.
Closing
You can add structured data in other formats too, although, Google recommends JSON+LD
.
You can also add the structured data in the page's body instead of head. You can even inject it dynamically, according to the documentation. My tests say otherwise but your results may vary.
I opted to initialise the blog data in the asyncData
method and insert the structured data into the head. Afterall, doing both is straightforward with Nuxt and Vue Meta.